- Go to the OmniCore API Key page
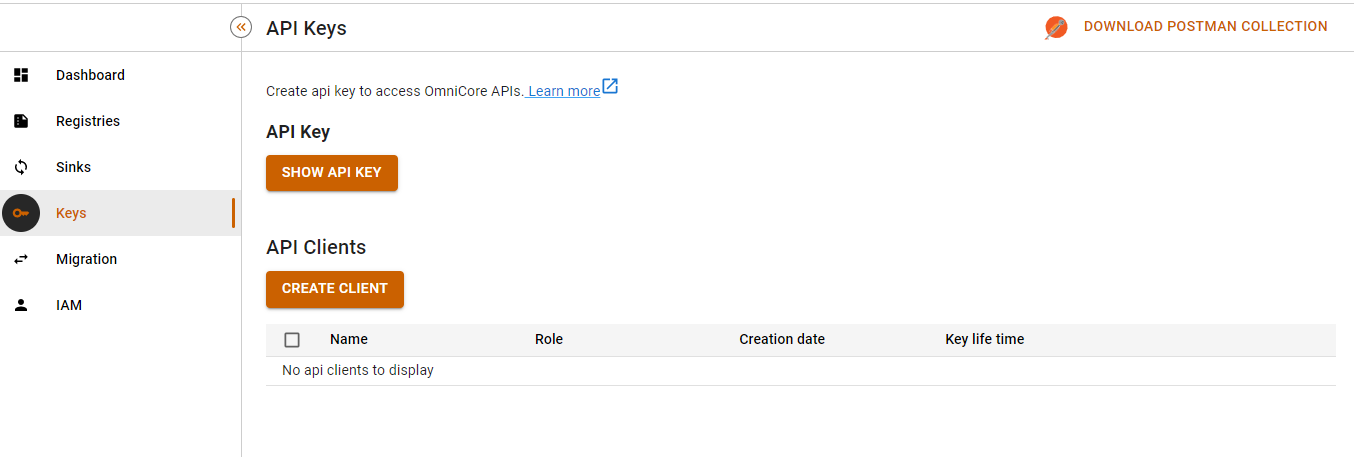
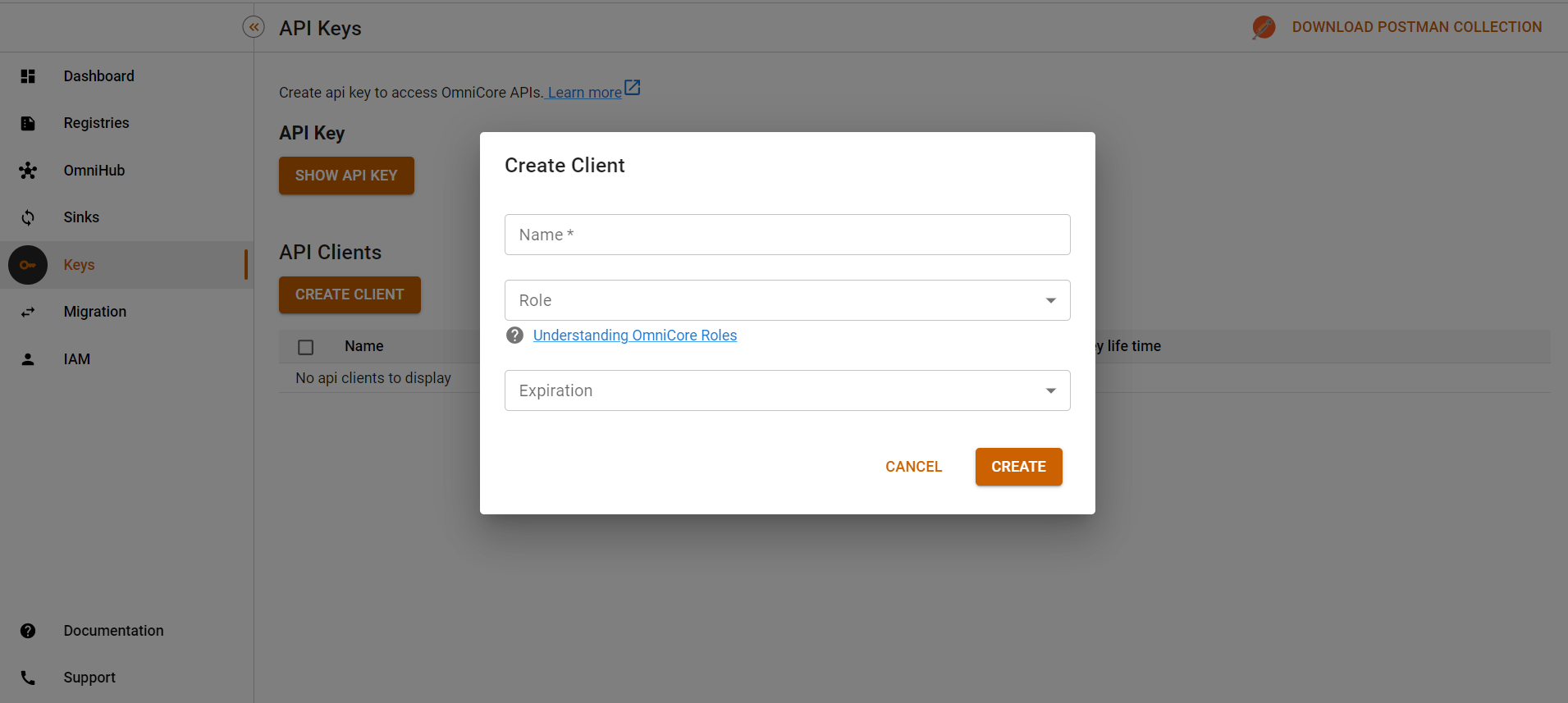
- Get Client ID and Client secret by clicking the SHOW KEY button.
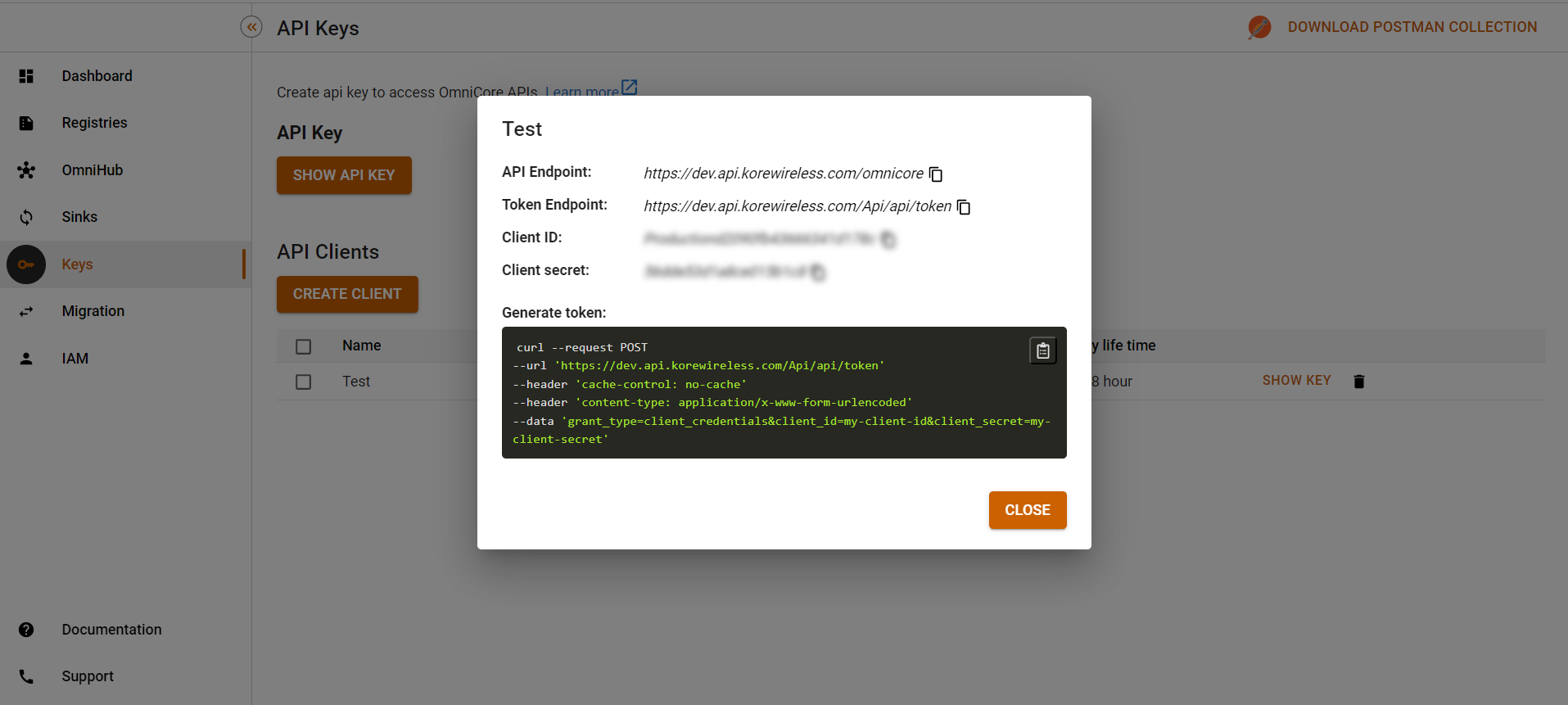
- Post the Client ID and Client Secret to the https://api.korewireless.com/Api/token endpoint for geting the API token.
cURL
curl --location --request POST 'https://api.korewireless.com/Api/token' \
--header 'cache-control: no-cache' \
--header 'content-type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'client_id=my-client-id' \
--data-urlencode 'client_secret=my-client-secret'
Node js
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.korewireless.com/Api/token',
'headers': {
'cache-control': 'no-cache',
'content-type': 'application/x-www-form-urlencoded'
},
form: {
'grant_type': 'client_credentials',
'client_id': 'my-client-id',
'client_secret': 'my-client-secret'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
Python
import requests
url = "https://api.korewireless.com/Api/token"
payload='grant_type=client_credentials&client_id=my-client-id&client_secret=my-client-secret'
headers = {
'cache-control': 'no-cache',
'content-type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.json()["access_token"])
- Use the API Key and API token to invoke OmniCore API
cURL
curl --location --request GET 'https://api.korewireless.com/omnicore/subscriptions/my-subscription-id/registries?pageNumber=1&pageSize=15' \
--header 'accept: application/json' \
--header 'authorization: Bearer API_TOKEN' \
--header 'x-api-key: MY_API_KEY'
Node js
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.korewireless.com/omnicore/subscriptions/my-subscription-id/registries?pageNumber=1&pageSize=15',
'headers': {
'accept': 'application/json',
'authorization': 'Bearer API_TOKEN',
'x-api-key': 'MY_API_KEY'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
Python
import requests
url = "https://api.korewireless.com/omnicore/subscriptions/my-subscription-id/registries?pageNumber=1&pageSize=15"
payload={}
headers = {
'accept': 'application/json',
'authorization': 'Bearer API_TOKEN',
'x-api-key': 'MY_API_KEY'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)